Understanding Average Stock Market Returns and Investment Strategies
What are penny stocks, and is it wise to invest in them?
How to install Tailwind CSS in React
GTA 6 Trailer 2025: Explore Vice City with Lucia - Everything We Know So Far
A Complete Overview of Web3 Wallets and How Does It Work?
Mastering the Inverse Head and Shoulders Pattern: A Comprehensive Guide
Smooth Scroll to Div using jQuery
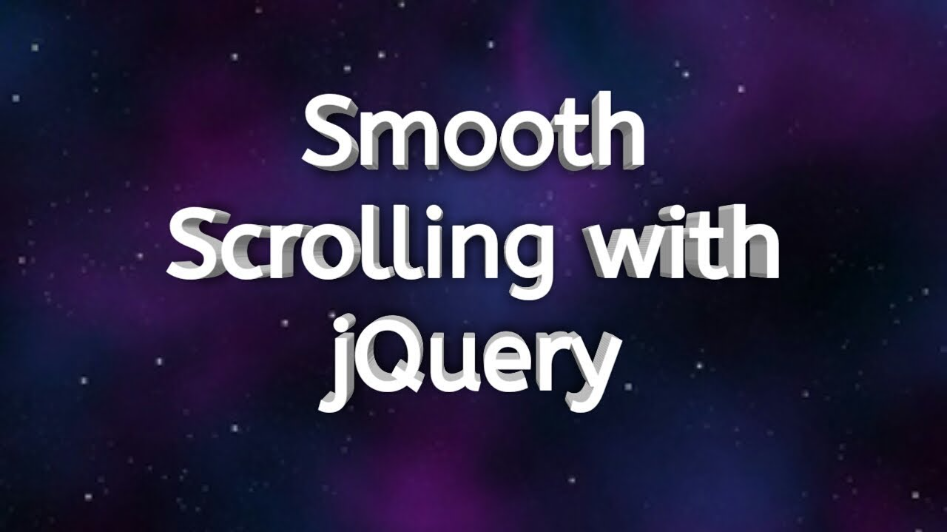
Smooth scrolling using jQuery enhances the user interface of web projects, reducing the effort required to navigate to specific sections of a page. By incorporating smooth scroll functionality, users can effortlessly reach desired portions of the page through anchor links or buttons.
Utilizing the jQuery scrollTop method simplifies the process of scrolling to a specific div element. To add a touch of animation to the page scroll, the jQuery animate() method can be employed. In this tutorial, we'll guide you through implementing smooth scrolling to a div using jQuery, eliminating the need for manual scrolling.
Scrolling to a Div
The Scroll to Div feature proves particularly beneficial for single-page websites, allowing users to navigate to specific sections seamlessly. The example code below demonstrates how to scroll to a div and jump to specific sections of the page by clicking on anchor links, all achieved with jQuery.
Smooth Scrolling Script:
Uses jQuery to enable smooth scrolling when clicking on navigation links. It targets all anchor (<a>) elements with an href attribute containing # (except for #) and adds a click event listener. When a link is clicked, it scrolls to the target section with a smooth animation.
The smooth scrolling is achieved through the jQuery animate function, scrolling to the target section's top position over 1000 milliseconds (1 second).
- The CSS styles mainly focus on adjusting font sizes for various elements.
- The navigation links in the #top div provide a quick way to navigate between sections.
- Sections 3 and 4 have a "BackToTop" link that uses the smooth scrolling script to scroll back to the top of the page.
- This code utilizes jQuery for simplicity. Ensure that the jQuery library is available, either by the provided CDN or by downloading and hosting it locally.
Recent Posts
Mastering the Inverse Head and Shoulders Pattern: A Comprehensive Guide
What are penny stocks, and is it wise to invest in them?
Understanding Average Stock Market Returns and Investment Strategies
Smooth Scroll to Div using jQuery
Back to Top Button using jQuery and CSS
How to install Tailwind CSS in React
How To Use the .htaccess File
What is a Hardware Wallet and How Does it Work?
A Complete Overview of Web3 Wallets and How Does It Work?
Create a Responsive Navbar using ReactJS
Express JS tutorial for beginners
Mastering the Inverse Head and Shoulders Pattern: A Comprehensive Guide
Create a Responsive Navbar using ReactJS
Express JS tutorial for beginners
You may also like…